编程界的小伙伴们,你们有没有想过,用C语言这样的“老古董”也能编写出精彩纷呈的游戏呢?没错,今天我就要带你走进C语言的奇妙世界,看看它是如何化身为游戏开发的大将的!
C语言的魅力:从“Hello World”到游戏世界
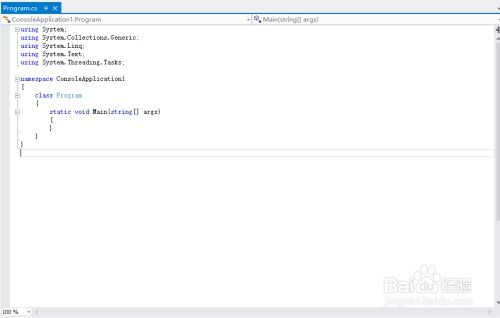
提起C语言,很多人可能会想到那些复杂的算法和严谨的逻辑。但你知道吗?C语言其实有着强大的生命力,它不仅能在系统编程、嵌入式开发等领域大放异彩,还能在游戏开发领域独树一帜。
C语言之所以能在游戏开发中占据一席之地,主要得益于以下几个特点:
1. 高效性:C语言编译后的程序运行速度快,内存占用小,这对于追求高性能的游戏来说至关重要。
2. 灵活性:C语言提供了丰富的库函数和扩展功能,可以轻松实现各种游戏效果。
3. 跨平台性:C语言编写的程序可以在多种操作系统上运行,这对于游戏开发者来说无疑是一个巨大的优势。
C语言游戏开发:从入门到精通
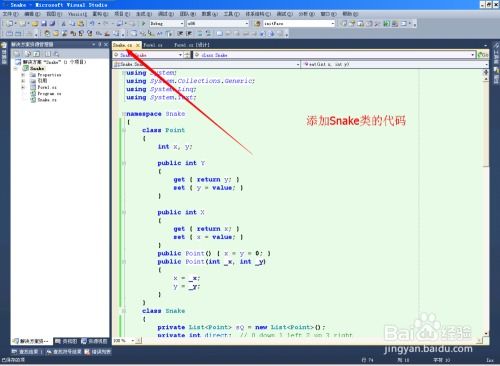
那么,如何用C语言编写游戏呢?下面,我就以几个简单的例子,带你一步步走进C语言游戏的世界。
1. 控制台游戏:从“猜数字”开始
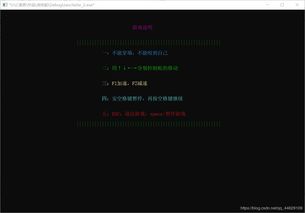
控制台游戏是C语言游戏开发的入门之作。我们可以通过简单的输入输出,实现一个“猜数字”游戏。
```c
include
include
include
int main() {
int number, guess;
srand(time(NULL));
number = rand() % 100 + 1;
printf(\猜一个1到100之间的数字:\
while (1) {
scanf(\%d\, &guess);
if (guess == number) {
printf(\恭喜你,猜对了!\
break;
} else if (guess < number) {
printf(\太小了,再试一次:\
} else {
printf(\太大了,再试一次:\
}
}
return 0;
2. 简单图形游戏:贪吃蛇
贪吃蛇是经典的图形游戏,用C语言也可以轻松实现。
```c
include
include
include
include
define WIDTH 20
define HEIGHT 20
int x, y, fruitX, fruitY, score;
int tailX[100], tailY[100];
int nTail;
enum eDirecton { STOP = 0, LEFT, RIGHT, UP, DOWN};
enum eDirecton dir;
void Setup() {
dir = STOP;
x = WIDTH / 2;
y = HEIGHT / 2;
fruitX = rand() % WIDTH;
fruitY = rand() % HEIGHT;
score = 0;
void Draw() {
system(\cls\);
for (int i = 0; i < WIDTH + 2; i++)
printf(\\);
printf(\\
for (int i = 0; i < HEIGHT; i++) {
for (int j = 0; j < WIDTH; j++) {
if (j == 0)
printf(\\);
if (i == y && j == x)
printf(\O\);
else if (i == fruitY && j == fruitX)
printf(\F\);
else {
int print = 0;
for (int k = 0; k < nTail; k++) {
if (tailX[k] == j && tailY[k] == i) {
printf(\o\);
print = 1;
}
}
if (!print) printf(\ \);
}
if (j == WIDTH - 1)
printf(\\);
}
printf(\\
}
for (int i = 0; i < WIDTH + 2; i++)
printf(\\);
printf(\\
printf(\Score: %d\
\, score);
void Input() {
if (_kbhit()) {
switch (_getch()) {
case 'a':
dir = LEFT;
break;
case 'd':
dir = RIGHT;
break;
case 'w':
dir = UP;
break;
case 's':
dir = DOWN;
break;
case 'x':
exit(0);
}
}
void Algorithm() {
int prevX = tailX[0];
int prevY = tailY[0];
int prev2X, prev2Y;
tailX[0] = x;
tailY[0] = y;
for (int i = 1; i < nTail; i++) {
prev2X = tailX[i];
prev2Y = tailY[i];
tailX